Definition
The Prototype design pattern is a creational pattern in object-oriented programming that allows you to create new objects by cloning existing ones.
This approach offers several advantages, including improved performance and code reusability.
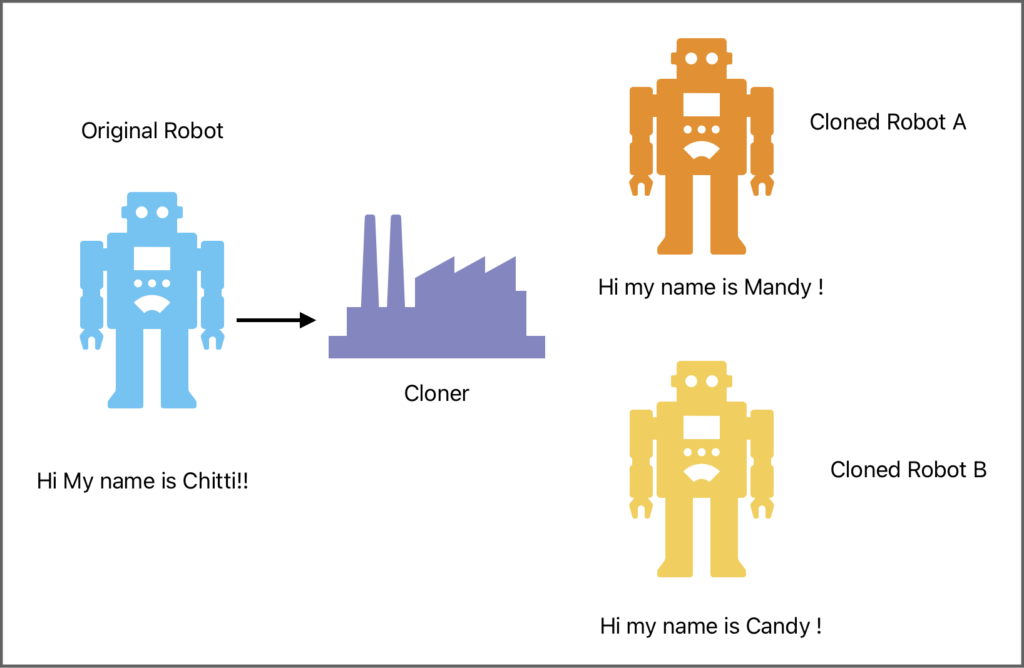
Purpose
The primary goal of the Prototype pattern is to:
- Reduce Object Creation Costs: By cloning existing objects, you can avoid the overhead associated with expensive initialization processes or complex object construction logic.
- Promote Reusability: Existing objects with desired properties can be easily reused as the basis for new objects.
- Simplify Customization: Clone objects and modify specific properties to create variations without affecting the original prototype.
Problem Statement
Imagine a scenario where your application creates complex game characters with intricate weapon and armor configurations. Each character creation involves substantial resource loading and customization logic. This approach has drawbacks:
- Performance Overhead: Repeating initialisation processes can slow the creation of multiple characters.
- Code Redundancy: If character creation logic involves complex steps, it might be duplicated for slightly different character types.
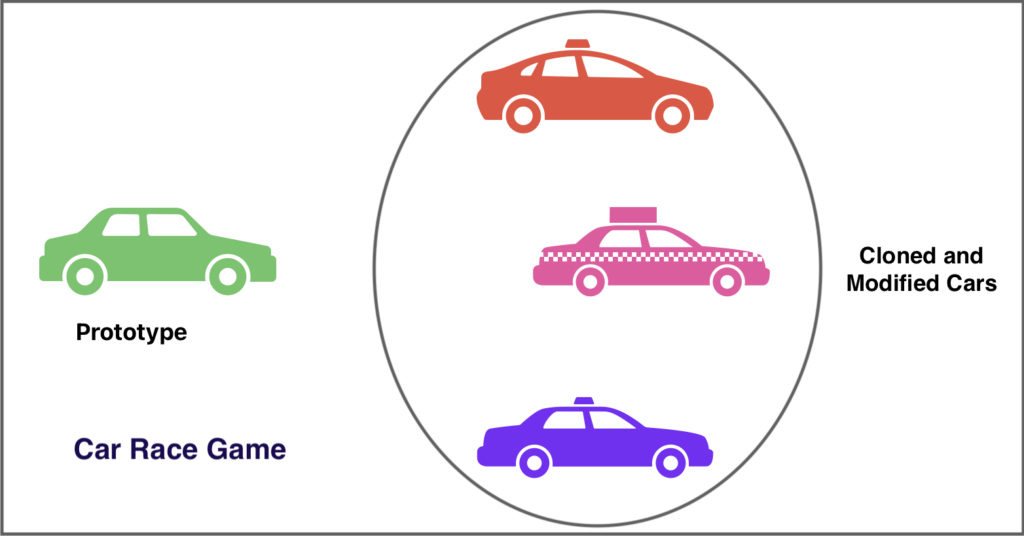
Solution: The Cloning Power
The Prototype pattern introduces a new layer – the Prototype interface or abstract class. This entity defines a method for cloning itself (e.g., clone()
).
Concrete classes implementing the prototype represent the specific object types you can create. Client code interacts with the prototype to obtain new objects through cloning.
Singleton Design Pattern In Java | Purpose | Implementation | Pros & Cons
Implementation of prototype design pattern in Java
Here’s an example implementation of the Prototype design pattern in Java:
// Prototype interface
interface Prototype extends Cloneable {
Prototype clone() throws CloneNotSupportedException;
}
// Concrete prototype class
class Employee implements Prototype {
private String name;
private int age;
private String department;
public Employee(String name, int age, String department) {
this.name = name;
this.age = age;
this.department = department;
}
@Override
public Prototype clone() throws CloneNotSupportedException {
return (Employee) super.clone();
}
@Override
public String toString() {
return "Employee [name=" + name + ", age=" + age + ", department=" + department + "]";
}
}
// Client code
public class PrototypeDemo {
public static void main(String[] args) throws CloneNotSupportedException {
Employee employee1 = new Employee("John", 30, "Engineering");
System.out.println("Original employee: " + employee1);
// Create a clone of the employee
Employee employee2 = (Employee) employee1.clone();
System.out.println("Cloned employee: " + employee2);
// Modify the original employee
employee1.setAge(35);
System.out.println("Modified original employee: " + employee1);
System.out.println("Cloned employee (unmodified): " + employee2);
}
}
In this example:
- We define an interface
Prototype
that declares aclone()
method. This interface extendsCloneable
because we’ll be using theObject.clone()
method to create clones. - We create a concrete class
Employee
that implements thePrototype
interface. Theclone()
method inEmployee
simply callssuper.clone()
to create a shallow copy of the object. - In the
main()
method of thePrototypeDemo
class, we create anEmployee
objectemployee1
. - We then create a clone of
employee1
by callingemployee1.clone()
and casting the result toEmployee
. - We modify the
age
of the originalemployee1
object and print out both the original and the cloned objects to demonstrate that the clone is a separate object and is unaffected by changes to the original.
When you run this code, you’ll see the following output:
Original employee: Employee [name=John, age=30, department=Engineering]
Cloned employee: Employee [name=John, age=30, department=Engineering]
Modified original employee: Employee [name=John, age=35, department=Engineering]
Cloned employee (unmodified): Employee [name=John, age=30, department=Engineering]
Builder Design Pattern In Java, Purpose, Implement, Use Cases, Pros & Cons
Pros and Cons
Pros
- Improved Performance: Cloning can be faster than creating complex objects from scratch.
- Code Reusability: Prototypes promote code reuse and reduce redundancy in object creation logic.
- Flexibility for Customization: Cloned objects can be easily modified to create variations.
Cons
- Increased Complexity: Introduces an additional layer of abstraction (prototype interface/class).
- Shallow Cloning Issues: By default, cloning might only copy object references, not the actual referenced objects. Deep cloning needs extra implementation.
When to Use the Prototype Pattern
- When object creation is expensive due to complex initialization or resource loading.
- When you need to create multiple objects with similar properties, but with minor variations.
- When a system needs to support the creation of new object types dynamically.
Where to Avoid the Prototype Pattern
- When dealing with simple objects with straightforward creation logic.
- When performance is not a critical concern, and the added complexity of the pattern outweighs the benefits.
Real-Life Examples
- Document Editing Software: Prototype patterns can create new documents by cloning existing templates, promoting consistency and reusability.
- Network Security Systems: Prototype patterns might be employed to create new network security policies by cloning existing ones and modifying specific rules.
Use Cases in Selenium Web Automation
The Prototype pattern can be useful in Selenium web automation to create reusable Page Object Models (POMs). By cloning a base POM class for specific pages, you can avoid code duplication and maintain consistent object interactions across different pages.
Use Cases in API Automation Using Rest Assured
In API automation with Rest Assured, the Prototype pattern can be applied to create reusable request objects with common base configurations. You can then clone these objects and modify specific parameters for different API calls.
Relationship with Other Patterns
The Prototype pattern can be combined with other patterns for more complex scenarios:
- Singleton Pattern: A Prototype can be used within a Singleton pattern to ensure only one instance of a complex object exists, but allow cloning for variations.
- Builder Pattern: When complex object creation involves many parameters, the Builder pattern can be used in conjunction with Prototype to simplify object cloning and customization.
Conclusion
The prototype design pattern in Java offers an efficient way to create new objects by cloning existing ones. This approach improves performance, promotes code reusability, and provides flexibility for customization.
However, it’s essential to consider the potential overhead of the additional layer of abstraction and potential deep cloning complexities.
By carefully evaluating your specific needs and weighing the pros and cons, you can determine if the Prototype pattern is the right choice for your project.
It shines in situations where object creation is expensive, code reusability is desired, and customization flexibility is valuable.