Interoduction to Design Patterns
What are Design Patterns?
In the realm of software development, design patterns serve as established solutions to frequently encountered programming challenges. They aren’t pre-written code snippets but function as blueprints or templates, offering a versatile approach to organizing code.
Here’s a detailed look at design patterns:
Benefits of Design Patterns:
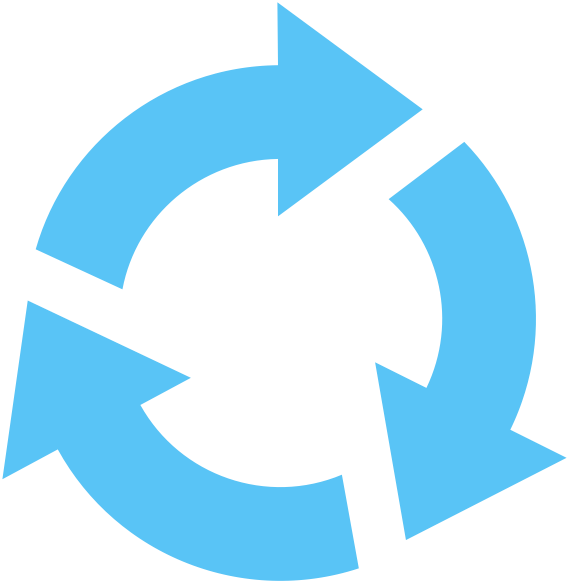
Reusability
Instead of starting from scratch each time, design patterns provide predefined solutions that can be adjusted and applied to tackle similar issues across various projects. This saves time and effort during development.
Flexibility
Design patterns serve as a solid groundwork, allowing for customization based on specific requirements. This strikes a balance between reusability and adaptability.
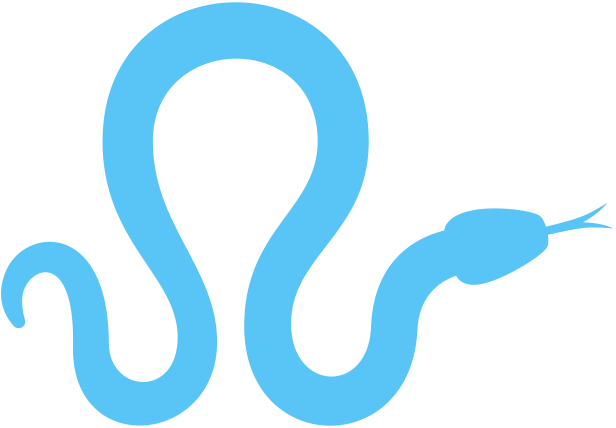
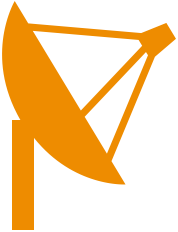
Communication
Utilizing common design patterns streamlines communication among developers. A shared understanding of these patterns enables them to comprehend each other’s code more effortlessly.
Maintainability
Code structured using design patterns tends to be more understandable and adaptable for future developers. The clarity of structure and adherence to established practices promote superior code organization.
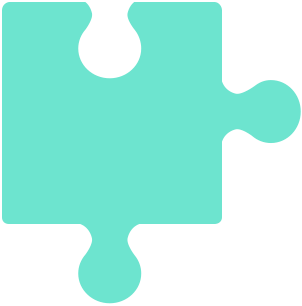
Types of Design Patterns
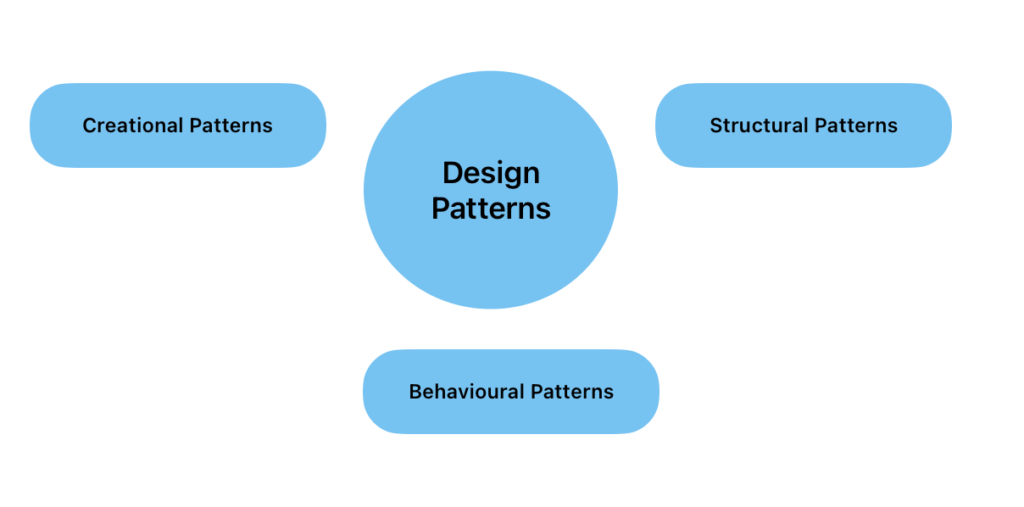
Design Pattern | Definition |
---|---|
Creational Patterns | These patterns focus on object creation, specifying how and when to create objects in a controlled and efficient manner. Example: Factory Pattern – This pattern centralizes object creation, allowing you to choose the specific type of object to create at runtime. |
Structural Patterns | These patterns deal with the structure of classes and objects, defining how they relate to each other and how to compose them to form larger structures. Example: Adapter Pattern – This pattern allows incompatible interfaces to work together by converting the interface of one object to another that the client expects. |
Behavioral Patterns | These patterns define how objects interact and communicate with each other, specifying algorithms and communication mechanisms for objects. Example: Observer Pattern – This pattern allows objects to subscribe to changes in another object (subject). When the subject’s state changes, all subscribed objects are notified. |
Java Example: Factory Pattern
Imagine you’re building a game and need to create different types of enemies: Orcs, Goblins, and Skeletons. Here’s a simplified example of the Factory Pattern in Java:
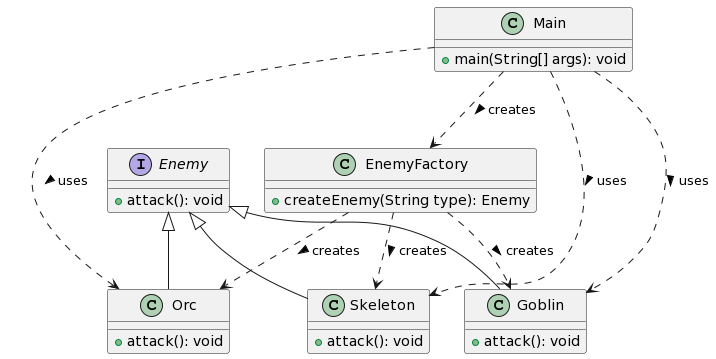
interface Enemy {
void attack();
}
class Orc implements Enemy {
@Override
public void attack() {
System.out.println("Orc swings axe!");
}
}
class Goblin implements Enemy {
@Override
public void attack() {
System.out.println("Goblin throws spear!");
}
}
class Skeleton implements Enemy {
@Override
public void attack() {
System.out.println("Skeleton shoots arrow!");
}
}
class EnemyFactory {
public Enemy createEnemy(String type) {
if (type.equals("orc")) {
return new Orc();
} else if (type.equals("goblin")) {
return new Goblin();
} else if (type.equals("skeleton")) {
return new Skeleton();
} else {
throw new IllegalArgumentException("Invalid enemy type");
}
}
}
public class Main {
public static void main(String[] args) {
EnemyFactory factory = new EnemyFactory();
Enemy enemy1 = factory.createEnemy("orc");
enemy1.attack(); // Prints: Orc swings axe!
Enemy enemy2 = factory.createEnemy("goblin");
enemy2.attack(); // Prints: Goblin throws spear!
}
}
This example demonstrates how the Factory Pattern provides a central location for enemy creation, making your code more flexible and easier to maintain. You can add new enemy types without modifying the main logic.
By understanding and applying design patterns, you can write cleaner, more maintainable, and reusable code, making you a more effective software developer.
Post Comment