What is JavaScriptExecutor?
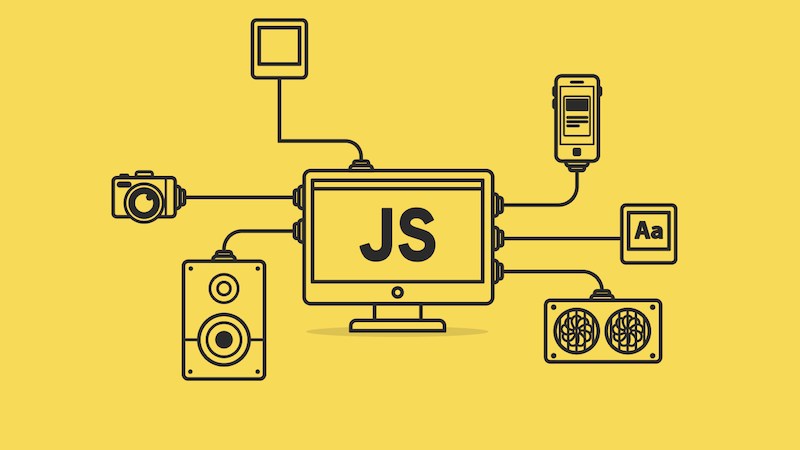
JavaScriptExecutor is an interface provided by Selenium WebDriver that allows you to execute JavaScript code in the context of the currently selected window or frame. It has two methods:
- executeAsyncScript(): This method executes the asynchronous JavaScript code in the context of the currently selected window or frame. An asynchronous JavaScript executed is a single thread while the rest of the page continuously parses, which enhances the performance.
executeScript()
: This method executes the JavaScript code in the context of the currently selected window or frame. The script runs as an anonymous function and the hand can return values.
The data types produced are:- WebElement
- List
- String
- Long
- Boolean
What is the Use of JavaScriptExecutor In Selenium?
JavaScriptExecutor can be used to do a variety of things, such as:
- Scroll to an element
- Get the value of an element
- Set the value of an element
- Click on an element
- Resize the window
- Scroll the page
- Inject CSS or JavaScript into the page
- Generate an alert
How to Use?
To use JavaScriptExecutor, you first need to get a reference to it. You can do this by casting the WebDriver object to a JavaScriptExecutor object. For example:
JavascriptExecutor js = (JavascriptExecutor) driver;
Once you have a reference to JavaScriptExecutor, you can use the executeScript()
or executeAsyncScript()
methods to execute JavaScript code.
Here is an example of how to use JavaScriptExecutor to scroll to an element:
js.executeScript("window.scrollTo(0, document.getElementById('elementId').offsetTop)");
This code will scroll the window to the top of the web element with the ID elementId
.
Selenium Top Interview Questions 2023
Examples of JavaScriptExecutor In Selenium
Horizontal Scroll
There exist various methods for horizontal scrolling, each applicable to different scenarios. Perhaps one of the following approaches might suit your needs.
Scenario: How to Scroll horizontally if Scroll Locator Exists
JavascriptExecutor jsExecutor = (JavascriptExecutor) driver;
jsExecutor.executeScript(String.format("arguments[0].scrollLeft+=%d", element.getLocation().getX() + 150) , element);
scrollLeft
Property: ThescrollLeft
property is a standard DOM property that represents the number of pixels an element’s content is scrolled horizontally. By incrementing thescrollLeft
property, you’re moving the content of the element to the right.- Element’s Location and Offset:
element
refers to a web element object that you want to horizontally scroll.element.getLocation().getX()
retrieves the X-coordinate of the element’s location on the page.- Adding
150
pixels to the element’s X-coordinate effectively scrolls the element 150 pixels to the right.
Scenario: How to Scroll horizontally if Scroll Locator doesn’t Exist
JavascriptExecutor jsExecutor = (JavascriptExecutor) driver;
jsExecutor.executeScript("window.scrollBy(6000,50);");
The window.scrollBy()
method is a built-in JavaScript method that scrolls the browser window by the specified amounts. In this case:
6000
represents the number of pixels to scroll horizontally to the right.50
represents the number of pixels to scroll vertically down.
Scroll Element Into View
Scenario: How to Scroll the element into view (To the top of the page)
JavascriptExecutor jsExecutor = (JavascriptExecutor) driver;
// Call JavaScript ScrollIntoView Fucntion to move element into View and later we can use selenium commands
jsExecutor.executeScript("arguments[0].scrollIntoView();", element);
scrollIntoView() Function:
- The
scrollIntoView()
function is a native JavaScript function that is supported by most modern browsers. - It ensures that the target element is brought into the visible area of the browser window by scrolling the page as needed.
- This can be particularly useful when working with elements that are not currently visible due to being located below or above the current view.
Scenario: How to Scroll Vertically if no web element exists for the scroller
In this case, we can use the javascript scrollBy
function
JavascriptExecutor jsExecutor = (JavascriptExecutor) driver;
jsExecutor.executeScript("window.scrollBy(50,6000);");
The window.scrollBy()
method is a built-in JavaScript method that scrolls the browser window by the specified amounts. In this case:
50
represents the number of pixels to scroll horizontally to the right.6000
represents the number of pixels to scroll vertically down.
Get Value Of Element
This code is to extract the current value of an input element (like a text field or text area) using JavaScript. This could be useful, for instance, if you need to verify or manipulate the value of the element.
// Create a JavaScriptExecutor instance
JavascriptExecutor jsExecutor = (JavascriptExecutor) driver;
// Use JavaScriptExecutor to get the value of the element
String elementValue = (String) jsExecutor.executeScript("return arguments[0].value;", element);
// Print the retrieved element value
System.out.println("Element Value: " + elementValue);
arguments[0]
refers to the first argument passed to the script, which is theelement
object you provide when calling theexecuteScript
method..value
accesses the “value” attribute of the element, which is used in various input elements like text fields and text areas.- The
element
mentioned in the script refers to a web element object whose “value” attribute you want to retrieve. The script takes the element as an argument. - The result of executing the JavaScript script is returned by the
executeScript
method. In this case, the expected result is a string (the value of the “value” attribute). The result is cast to aString
using(String)
.
How to Click on Element
The purpose of this code is to programmatically trigger a click action on a web element.
This can be useful when you want to interact with elements that might not respond properly to WebDriver’s built-in click()
method, or when you need to manipulate the page using JavaScript.
// Create a JavaScriptExecutor instance
JavascriptExecutor js = (JavascriptExecutor) driver;
// Use JavaScriptExecutor to click on the element
js.executeScript("arguments[0].click();", element);
arguments[0]
refers to the first argument passed to the script, which is theelement
object you provide when calling theexecuteScript
method..click()
simulates a mouse click on the element, just as if a user clicked on it.- The
element
mentioned in the script refers to a web element object that you want to simulate clicking on. The script takes the element as an argument.
Click On Element Using querySelector()
JavascriptExecutor jsExecutor = (JavascriptExecutor) driver;
// click using query Selector function
jsExecutor.executeScript("document.querySelectorAll('#username').forEach(el=>el.click())");
Get Text Of an Element Using Xapth
Scenario: If Selenium commands are not working anyhow or are not able to read the text content of an element. Then using the below code shows how we can use Xpath.
The purpose of this code is to dynamically retrieve the text content of an element on the web page using an XPath expression. This can be useful when you need to verify or work with the content of a specific element.
final String EMAIL_XPATH = "//div[@id='email']";
JavascriptExecutor jsExecutor = (JavascriptExecutor) driver;
String textContent = jsExecutor.executeScript(format("return document.evaluate(\"%s\", document, null, XPathResult.FIRST_ORDERED_NODE_TYPE, null).singleNodeValue.textContent", EMAIL_XPATH)).toString();
Breakdown Of the above Code
- Using
document.evaluate()
: Thedocument.evaluate()
method is a built-in JavaScript function that is used to evaluate an XPath expression in the context of the provided document. It allows you to search for elements based on the XPath expression. - Dynamic XPath Expression: The code uses
format
to include a dynamic XPath expression within the JavaScript code. TheEMAIL_XPATH
variable is expected to hold the actual XPath expression that targets the desired element. - XPathResult.FIRST_ORDERED_NODE_TYPE: The
XPathResult.FIRST_ORDERED_NODE_TYPE
constant specifies that you want to retrieve the first matching node from the result of the XPath evaluation. - Retrieving Text Content: The JavaScript code uses
.singleNodeValue.textContent
to extract the text content of the matched element. - Casting to String: The result of executing the JavaScript code using
executeScript
is expected to be a JavaScript string representing the text content of the element. The result is cast to aString
using.toString()
.
Update Some attributes at runtime
The purpose of this code is to programmatically set the value of a search input element to 'Today Weather'
. This simulates a user entering text into a search box.
JavascriptExecutor jsExecutor = (JavascriptExecutor) driver;
jsExecutor.executeScript("document.querySelector(\"input[type='search']\").value='Today Weather'");
document.querySelector()
: Thedocument.querySelector()
method is a built-in JavaScript function that uses a CSS selector to find the first matching element in the document. In this case, it’s being used to find an<input>
element with the attributetype
set to'search'
.- Assigning a Value: After locating the search input element,
.value
is used to set the value attribute of the element. In this case, the value'Today Weather'
is assigned to the search input.
Use Xapth If CSS is not working
Using evaluate you can use Xpath. Generally, we prefer CSS selector but if nothing is working then in that case this option will help you.
final String PASSWORD_XPATH = "//div[@id='password']";
JavascriptExecutor jsExecutor = (JavascriptExecutor) driver;
String value = jsExecutor.executeScript(format("return document.evaluate(\"%s\", document, null, XPathResult.FIRST_ORDERED_NODE_TYPE, null).singleNodeValue.value", PASSWORD_XPATH)).toString();
Related FAQs:
- How To Scroll a Page Using Selenium WebDriver?
- How to Page scroll up or down in Selenium WebDriver?
- How To Perform Scroll Operations Using JavaScript?
- How to Scroll into view in Selenium Webdriver?
- What is JavaScriptExecutor in Selenium?
- Explain JavaScriptExecutor in Selenium with an Example.
You can find answers of all these questions in the above article. Thanks for reading!!!