JavaScript Executor in Selenium Python – Use with Examples
JavaScript Executor in Selenium Python
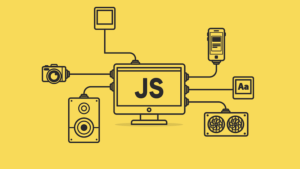
When it comes to automating WEB applications, Selenium stands out as a highly popular tool. Yet, there are instances where the built-in functions come up short in handling certain scenarios. Consider situations like:
- Scrolling to a specific element.
- Retrieving the value of an element.
- Setting the value of an element.
- Clicking on a designated element.
- Resizing the application window.
- Page scrolling actions.
- Injecting CSS or JavaScript code into the page.
- Triggering an alert.
These examples underscore the challenges one might encounter while employing Selenium for actions. However, a remedy exists. Enter the concept of the ‘JavaScript Executor in Selenium Python.’ for Python binding.
Similarly, we have ‘JavaScriptExecutor in Selenium‘.
Unveiling a proficient approach, it’s worth noting that Selenium harnesses JavaScript in its underlying operations. To address these limitations, Selenium furnishes the JavaScript Executor interface, which empowers users to execute these actions via JavaScript.
This invaluable interface remains universally accessible across all language bindings supported by Selenium.
How to Use JavaScript Executor in Selenium Python
Utilizing the JavaScript Executor in Python with Selenium involves a straightforward process. This capability empowers you to execute JavaScript code within the context of the web page being automated. Here’s a step-by-step guide:
The JavaScriptExecutor in Selenium Python is a class that allows you to execute JavaScript code in the current window or frame. This can be useful for a variety of tasks, such as:
- Getting or setting the value of a web element
- Scrolling to a particular location on the page
- Simulating user actions, such as clicking or hovering over an element
- Accessing the DOM (Document Object Model)
To use the JavaScriptExecutor, you first need to get a reference to it from the WebDriver object. Once you have a reference, you can use the executeScript()
method to execute a JavaScript snippet. The executeScript()
method takes two arguments:
1. Import Dependencies
First, ensure you have Selenium installed. You can install it using the following command:
pip install selenium
Then, import the necessary libraries in your Python script:
from selenium import webdriver
2. Create WebDriver Instance
Set up a WebDriver instance (e.g., ChromeDriver) to interact with the browser:
driver = webdriver.Chrome() # You can use other browsers like Firefox as well
3. Navigate to a Web Page
Use the WebDriver instance to navigate to the desired web page:
driver.get("https://www.example.com")
4. Execute JavaScript
To use the JavaScript Executor, call the execute_script()
method on the WebDriver instance. Pass the JavaScript code you want to execute as an argument. Here’s an example of scrolling to the bottom of the page:
# Scroll to the bottom of the page
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
5. Perform Other Actions
You can perform various operations using JavaScript, such as interacting with elements, modifying attributes, or even handling alerts.
For example:
# Clicking an element using JavaScript
element = driver.find_element_by_id("element_id")
driver.execute_script("arguments[0].click();", element)
6. Close the Browser
After you’re done, don’t forget to close the browser window.
driver.quit()
Putting it all together, here’s a complete example:
from selenium import webdriver
# Create a WebDriver instance
driver = webdriver.Chrome()
# Navigate to a web page
driver.get("https://www.example.com")
# Execute JavaScript to scroll to the bottom of the page
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
# Find an element and click it using JavaScript
element = driver.find_element_by_id("element_id")
driver.execute_script("arguments[0].click();", element)
# Close the browser window
driver.quit()
Remember that the JavaScript code you use within execute_script()
should be valid JavaScript syntax and compatible with the context of the web page you are interacting with.
I hope you liked this article on JavaScript Executor in Selenium Python and its use with proper examples.
If you want to see different examples of using javascript in selenium then you can go into this blog post javascript executor in selenium using java. This article is based on JAVA but you can follow internal javascript code which will be the same in Java and Python Automation.
If you’re interested in exploring various instances of employing JavaScript within Selenium, you can refer to the blog post titled ‘JavaScript Executor in Selenium using Java.‘ While the article pertains to Java, you can adopt the internal JavaScript code interchangeably for both Java and Python automation.
Don’t forget to share this article with the testing Communities on different Social Platform 🙂 🙂
Post Comment